Home » .NET Development » Parallel ForEach Loop in C# to Improve App Performance
Parallel ForEach Loop in C# to Improve App Performance

In this article, we’ll learn how we can boost the performance of the application using parallel ForEach loop in C# programming tricks of
Prerequisites:
- Prior knowledge of C#
- Visual Studio Code
Step-by-Step Tutorial of Parallel Processing in C# App to Improve Performance
Step 1: Create Console Application Project
By selecting create new project -> under Visual C# section -> Select Console App and create new project.
Step 2: Add any list in program.cs
- After creating new project add any list under List<string>.
- You can add any object naming list under it like countries name list, animals name list, Car manufacturing companies name list, etc.
- Alternatively, you can fetch it from database and can easily add to list of can be use directly.
- Here we are using country list for check that how parallel processing increase performance by applying on logic.
Step 3: Now it’s time to check performance by applying ForEach looping condition on list
Here, we are going to print country list on console screen by using ForEach loop.
if (lstCountries.Count > 0)
{
Console.WriteLine("Foreach loop start time : " + DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss.fff", CultureInfo.InvariantCulture));
foreach (var item in lstCountries)
{
Console.WriteLine(item.name);
}
Console.WriteLine("Foreach loop end time : " + DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss.fff", CultureInfo.InvariantCulture));
}
Also we will print process start time and process end time so we can compare normal foreach loop with parallel logic output time.
So after run of console app in output foreach loop start at 06:562 and complete it’s process on 06:679 which takes total 117 Milliseconds for print whole list of countries.
Step 4: Now applying looping with Parallel ForEach concept
- For applying parallel, use statement with “Parallel.Foreach” by using “System.Threading.Tasks” namespace.
Console.WriteLine("Parallel Foreach loop start time : " + DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss.fff", CultureInfo.InvariantCulture));
Parallel.ForEach(lstCountries, (country) =>
{
Console.WriteLine(country.name);
});
Console.WriteLine("Parallel Foreach loop end time : " + DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss.fff", CultureInfo.InvariantCulture));
- Above code showing that how can we apply or replace ForEach with parallel.foreach statement.
- In above Images, we can see in output screen parallel foreach loop starts at 06:681 and complete it’s process on 06:791 so almost it takes 110 milliseconds for print whole list of countries.
Step 5: Comparison of Loop Processing: ForEach Vs Parallel ForEach
- We have only 250 country name in our list and in comparison as we can see that normal foreach loop takes total 117 milliseconds for print country name in console app.
- However Parallel ForEach take 110 milliseconds for print same list on console screen.
Parameters | ForEach Loop | Parallel ForEach Loop |
Time Taken | 117 Milliseconds | 110 Milliseconds (Faster) |
Processing Performance | Run on Single Thread and Follow Sequential Processing | Run on Multiple Threads and Follow Parallel Processing |
When To Use | Small Data Task inside loop | Complex Long List Processing Task |
FAQ about Usage
When should we use ForEach or Parallel.ForEach loop?
If there is small data task inside loop then should use foreach loop. However if loop contains long list of items and contains complex processing task then should use parallel.foreach loop.
That’s it for now. Stay Connected for more tutorials, until than Happy Coding…
Our developers are always happy to assist you. To turn your business ideas into beautiful reality you can hire .NET developer with our easy hiring model.
Related C# MVC Development Resource
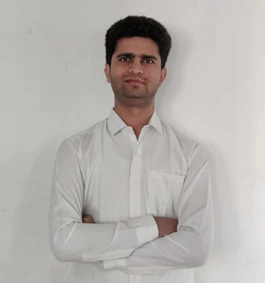
With over 10 years of experience in Tech industry at Samarpan Infotech with architect system, problem solving and creativity. "Today is the only day. Yesterday is gone".